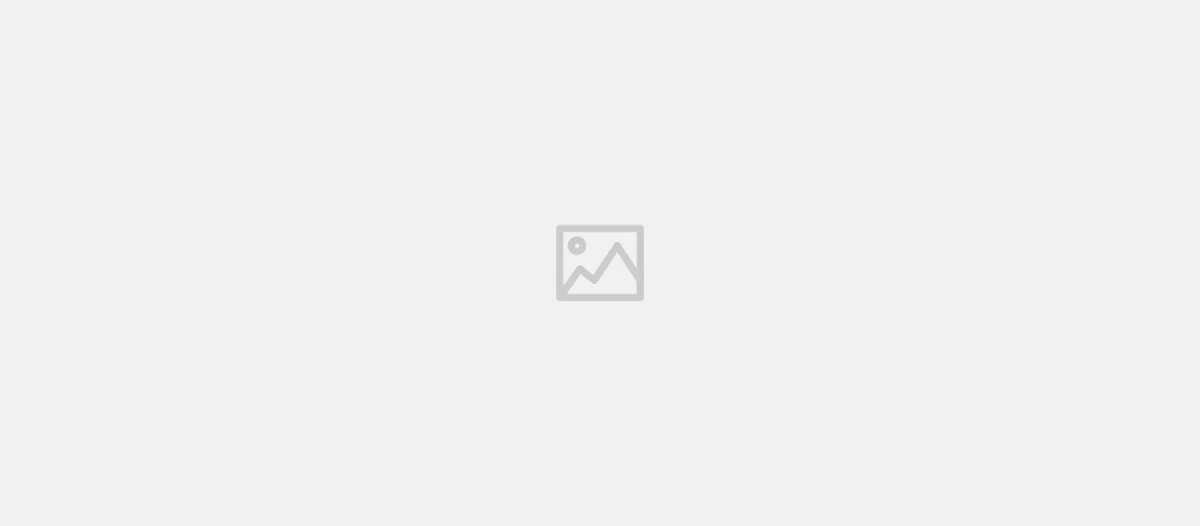
Writing Your First Code on a Raspberry Pi
- Solomon Ankomah
- How to
- 07 Dec, 2024
The Raspberry Pi is a versatile, credit-card-sized computer designed for learning, experimenting, and building projects. If you’re new to the Raspberry Pi, writing your first code can be an exciting step into the world of programming and electronics. This guide will walk you through the process of setting up your Raspberry Pi and writing your first program.
What You’ll Need
To get started, ensure you have the following:
- Raspberry Pi: Any model (e.g., Raspberry Pi 4, 3, Zero).
- MicroSD Card: At least 16GB, preloaded with Raspberry Pi OS or installable via Raspberry Pi Imager.
- Power Supply: A compatible power adapter for your Raspberry Pi model.
- Monitor, Keyboard, and Mouse: To interact with the Raspberry Pi (or use SSH/VNC for remote access).
- Internet Connection: For updates and installing software.
Note: If you haven’t set up your Raspberry Pi yet, check out this guide on setting up your Raspberry Pi for detailed instructions.
Step 1: Write a Simple Python Script
Python comes pre-installed on Raspberry Pi OS, making it an ideal language for beginners.
-
Open a Text Editor:
- For GUI: Open the Thonny Python IDE from the Raspberry Pi menu (Programming section).
- For CLI: Use the
nano
editor in the terminal.-
nano hello.py
-
-
Write Your Code: In Thonny or the terminal, write the following Python script:
print("Hello, Raspberry Pi!")
-
Save Your Script:
- In Thonny: Click
File > Save
, name ithello.py
, and save it in your home directory. - In
nano
: PressCtrl+O
, typehello.py
, press Enter, thenCtrl+X
to exit.
- In Thonny: Click
-
Run Your Script: Open the terminal and execute the script:
python3 hello.py
You should see:
Hello, Raspberry Pi!
Step 2: Control GPIO Pins (Optional)
If you want to interact with electronics like LEDs, you can use the Raspberry Pi’s GPIO pins.
Example: Blink an LED
-
Components Needed:
- 1 LED
- 1 Resistor (330Ω or 220Ω)
- Jumper wires
- Breadboard
-
Connect the Circuit:
- Connect the longer leg of the LED (anode) to GPIO pin 18.
- Connect the shorter leg (cathode) to a resistor, then to a ground (GND) pin.
-
Install the RPi.GPIO Library:
pip3 install RPi.GPIO
-
Write the Code: Create a new script
blink.py
with the following code:import RPi.GPIO as GPIO import time # Set up GPIO GPIO.setmode(GPIO.BCM) GPIO.setup(18, GPIO.OUT) # Blink the LED try: while True: GPIO.output(18, GPIO.HIGH) time.sleep(1) # 1 second on GPIO.output(18, GPIO.LOW) time.sleep(1) # 1 second off except KeyboardInterrupt: GPIO.cleanup()
-
Run the Script:
python3 blink.py
Watch your LED blink on and off!
Step 3: Explore More Projects
-
Networking:
- Build a web server using Flask.
- Control GPIO pins via a web interface.
-
Sensors:
- Read temperature and humidity using a DHT11 sensor.
- Use an ultrasonic sensor for distance measurement.
-
Media:
- Create a music player using Python and VLC.
- Set up a media server with Plex or Kodi.
-
Automation:
- Automate tasks with crontab and Python scripts.
- Build a smart home hub using MQTT and Raspberry Pi.
Debugging Tips
- Syntax Errors: Double-check your code for typos.
- Module Not Found: Install missing libraries with
pip3 install <library-name>
. - GPIO Errors: Ensure you run scripts with
sudo
when accessing GPIO pins. - Logs: Use
print()
statements to debug your code.
Conclusion
Writing your first code on a Raspberry Pi is the first step in an exciting journey into programming and hardware projects. With Python and the Raspberry Pi’s GPIO capabilities, the possibilities are endless. Experiment, learn, and build—the Raspberry Pi community is vast and always ready to help. Happy coding!