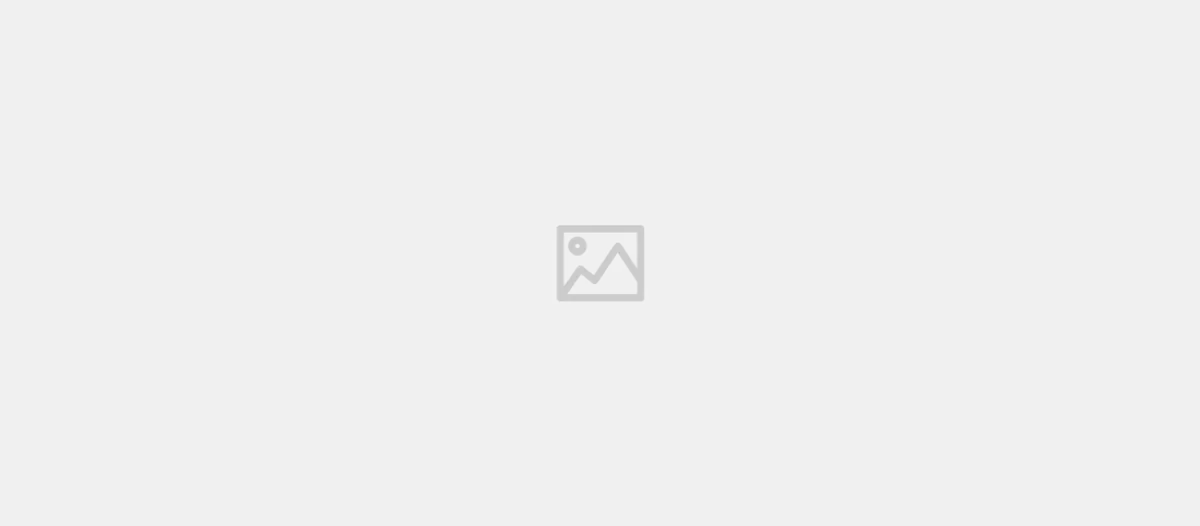
Getting Started with ESP32
- Solomon Ankomah
- Getting Started
- 01 Dec, 2024
The ESP32 is a powerful and versatile microcontroller, widely used for IoT (Internet of Things) applications due to its low cost, high processing power, built-in Wi-Fi, and Bluetooth capabilities. In this guide, we’ll walk you through setting up the ESP32 with the Arduino IDE, which is a beginner-friendly platform for programming microcontrollers.
Prerequisites
Before getting started, make sure you have the following:
- ESP32 Development Board: Popular options include ESP32 DevKit V1 or NodeMCU ESP32.
- USB Cable: A micro-USB or USB-C cable, depending on your ESP32 board.
- Computer: Windows, macOS, or Linux.
- Arduino IDE: Installed on your computer. You can download it from the official Arduino website.
Step 1: Install the Arduino IDE
- Download and Install: Go to the Arduino IDE download page and download the appropriate version for your operating system. Follow the installation instructions.
- Launch the IDE: Open the Arduino IDE after installation.
Step 2: Add ESP32 Board Support
The Arduino IDE does not come with built-in support for ESP32, so you’ll need to add it manually.
- Open Preferences:
- Go to File > Preferences (Windows/Linux) or Arduino > Preferences (macOS).
- Add Additional Board Manager URL:
- In the “Additional Board Manager URLs” field, paste the following URL: https://raw.githubusercontent.com/espressif/arduino-esp32/gh-pages/package_esp32_index.json
- If there are already URLs in the field, separate them with a comma.
- Open Boards Manager:
- Go to Tools > Board > Boards Manager.
- Search for “ESP32” and click “Install” for the esp32 by Espressif Systems package.
- Select the ESP32 Board:
- Go to Tools > Board > ESP32 Arduino and select your specific ESP32 board (e.g., “ESP32 Dev Module”).
Step 3: Install USB-to-Serial Drivers (If Necessary)
Some ESP32 boards require USB-to-Serial drivers to communicate with your computer. Common drivers include:
- CP210x: For boards using the Silicon Labs CP2102 chip.
- CH340: For boards using the CH340 chip.
Installation Steps:
- Identify the USB-to-Serial chip on your board (usually mentioned in the product description or printed on the board).
- Download the appropriate driver from the manufacturer’s website:
- Install the driver and restart your computer if required.
Step 4: Connect the ESP32 to Your Computer
- Plug the ESP32 board into your computer using the USB cable.
- Open the Arduino IDE and go to Tools > Port. Select the port that corresponds to your ESP32.
Step 5: Write Your First Program
To verify that everything is set up correctly, upload a simple “Blink” program to your ESP32.
Example Code:
void setup() {
// Initialize the built-in LED pin as an output
pinMode(2, OUTPUT);
}
void loop() {
digitalWrite(2, HIGH); // Turn the LED on
delay(1000); // Wait for 1 second
digitalWrite(2, LOW); // Turn the LED off
delay(1000); // Wait for 1 second
}
Steps to Upload:
- Copy the code above into the Arduino IDE.
- Click on the Verify button (checkmark icon) to compile the code.
- Click on the Upload button (arrow icon) to upload the code to the ESP32.
- Open the Serial Monitor (Tools > Serial Monitor) to check for any messages. Set the baud rate to 115200 if needed.
Step 6: Troubleshooting
If you encounter issues, try the following:
- Check Connections: Ensure the USB cable is properly connected and functional.
- Select the Correct Port: Go to Tools > Port and ensure the correct COM port is selected.
- Press the Boot Button: Some ESP32 boards require you to hold the “BOOT” button while uploading code.
- Reinstall Drivers: Verify that the USB-to-Serial drivers are correctly installed.
- Check Board Selection: Ensure you have selected the correct ESP32 board in Tools > Board.
Step 7: Explore More Features
Once the basic setup is complete, you can explore advanced features like:
- Wi-Fi Connectivity:
- Connect your ESP32 to a Wi-Fi network using the built-in Wi-Fi module.
- Bluetooth Communication:
- Use Bluetooth to communicate with smartphones or other devices.
- Sensors and Actuators:
- Interface the ESP32 with sensors (e.g., temperature, humidity) and actuators (e.g., relays, motors).
- IoT Platforms:
- Send data to cloud platforms like ThingSpeak, Blynk, or Firebase.
Conclusion
The ESP32 is a powerful microcontroller that can bring your IoT projects to life. With the Arduino IDE, setting it up and programming it becomes straightforward, even for beginners. Once you’ve mastered the basics, you can dive into more complex projects and fully leverage the capabilities of the ESP32.
Happy tinkering!