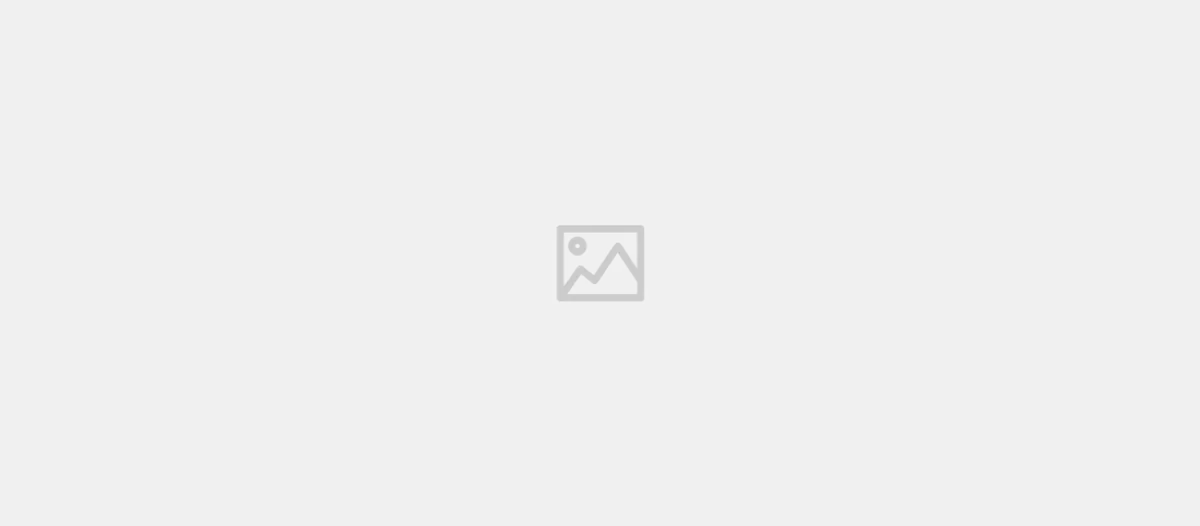
Getting Started with STM32
- Solomon Ankomah
- Getting Started
- 04 Dec, 2024
STM32 microcontrollers, developed by STMicroelectronics, are popular for their high performance, versatility, and extensive ecosystem of development tools. Whether you’re a beginner or an experienced embedded systems engineer, getting started with STM32 can be both exciting and rewarding. This guide provides a detailed walkthrough to help you set up and start working with STM32 microcontrollers.
Why Choose STM32?
STM32 microcontrollers are based on the ARM Cortex-M series, offering a wide range of features:
- Scalability: Available from low-power to high-performance families.
- Peripheral Integration: Includes UART, SPI, I2C, ADC, DAC, timers, and more.
- Low Power Consumption: Ideal for battery-powered devices.
- Robust Ecosystem: Supported by ST’s HAL (Hardware Abstraction Layer), STM32CubeMX, and community-driven resources.
- Wide Availability: Suitable for applications like IoT, automotive, industrial automation, and consumer electronics.
Step 1: Choosing the Right STM32 Board
STM32 comes in various families, such as:
- STM32F: General-purpose microcontrollers.
- STM32L: Low-power variants for energy-sensitive applications.
- STM32G: High-performance and cost-efficient.
- STM32H: High-performance and advanced features.
- STM32WB: With wireless connectivity (Bluetooth, Zigbee).
For beginners, the STM32F103 (Blue Pill) or NUCLEO boards are great starting points.
Step 2: Setting Up Your Development Environment
1. Software Requirements
To program and debug STM32 microcontrollers, you’ll need:
- STM32CubeIDE: An all-in-one development tool provided by STMicroelectronics.
- Download from STMicroelectronics.
- STM32CubeMX: A graphical tool to configure peripherals and generate initialization code.
- Optional Tools:
- Keil uVision or IAR Embedded Workbench (proprietary IDEs).
- Open-source tools like PlatformIO or Eclipse with STM32 plugins.
2. Hardware Requirements
- An STM32 development board (e.g., NUCLEO, DISCOVERY, or Blue Pill).
- Debugging tools:
- Integrated debugger on NUCLEO boards.
- ST-LINK V2 or J-Link for other boards.
- USB cable to connect the board to your PC.
Step 3: Creating Your First STM32 Project
1. Install STM32CubeIDE
- Download and install STM32CubeIDE from ST’s official website.
- Launch the IDE and configure your workspace.
2. Start a New Project
- Open STM32CubeIDE and click on “File > New > STM32 Project.”
- Use the board selector to choose your STM32 microcontroller or development board.
- Click “Next” and configure your project name and location.
- STM32CubeIDE will auto-generate a basic project template.
3. Configure Peripherals Using STM32CubeMX
- Use the graphical interface to enable peripherals like GPIO, UART, I2C, SPI, etc.
- Set clock configurations to match your application needs.
- Generate the initialization code by clicking on “Generate Code.”
4. Write Your Code
- Navigate to the
main.c
file in the “Core/Src” folder. - Add your application-specific logic.
Example: Blinking an LED on a GPIO pin:
#include "main.h"
int main(void) {
HAL_Init();
SystemClock_Config();
/* Configure GPIO pin */
__HAL_RCC_GPIOC_CLK_ENABLE();
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = GPIO_PIN_13;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(GPIOC, &GPIO_InitStruct);
while (1) {
HAL_GPIO_TogglePin(GPIOC, GPIO_PIN_13);
HAL_Delay(500);
}
}
5. Compile and Debug
- Click on the “Build” button to compile the code.
- Use the “Debug” button to flash and debug your application on the board.
Step 4: Exploring Advanced Features
Once you’ve mastered the basics, explore the advanced features of STM32:
1. RTOS (Real-Time Operating System)
- STM32CubeIDE supports FreeRTOS for multitasking.
- Add FreeRTOS middleware via STM32CubeMX.
2. Communication Protocols
- Experiment with UART, I2C, SPI, CAN, and USB for interfacing with external devices.
3. Low-Power Modes
- Use STM32’s power management features to optimize energy consumption.
4. Custom Bootloaders
- Implement custom bootloaders for advanced firmware upgrade mechanisms.
5. Wireless Communication
- Use STM32WB for Bluetooth or Zigbee applications.
Debugging and Troubleshooting Tips
-
Common Issues:
- Incorrect clock configuration.
- Improper peripheral initialization.
- Debugging connection errors.
-
Troubleshooting Tools:
- Use the “System Viewer” in STM32CubeIDE for debugging.
- Analyze signals with an oscilloscope or logic analyzer.
-
Community Support:
- Refer to the STM32 Community Forum.
- Explore open-source projects on GitHub.
Conclusion
Getting started with STM32 microcontrollers can seem daunting, but with the right tools and a structured approach, you’ll be building impressive projects in no time. By mastering STM32CubeIDE and understanding the hardware’s capabilities, you’re set to create innovative applications for a wide range of industries. Happy coding!